This article demonstrates how to provide suggestions to users using autocomplete within jQuery. Included are code and easy examples to follow.
The autocomplete widget we’ll be using is found within the jQuery UI library. This article will explain how to use the autocomplete with code blocks, configuration settings, and methods below. So if you’re ready, here we go!
Contents
- What is jQuery Autocomplete
- Installing jQuery Autocomplete
- Autocomplete Methods
- Usage and Example
- Final Words
What is jQuery Autocomplete
Autocomplete is a jQuery library that provides suggestions while writing in an input or text field. These suggestions speed up the interaction time between the user and the web page. For this reason, while developing a web page with form inputs, autocomplete can be a great idea to improve the design and usability if implemented correctly. So, in summary, the jQuery Autocomplete library provides an autocomplete widget to make it easier and faster for users to write by giving a list of typing suggestions in a text box.
Installing the Autocomplete Libary
The following CSS and JavaScript files must be added to a web page to use the autocomplete library. For the jQuery library, we need to place the script tag in the page’s header.
<script src="https://code.jquery.com/jquery-3.6.0.js"></script>
Addionally we will need the jQuery UI library and the related CSS files.
<link rel="stylesheet" href="//code.jquery.com/ui/1.13.1/themes/base/jquery-ui.css">
<script src="https://code.jquery.com/ui/1.13.1/jquery-ui.js"></script>
Once all three resources are included, your web page header should look something like this.
<head>
<script src="https://code.jquery.com/jquery-3.6.0.js"></script>
<link rel="stylesheet" href="//code.jquery.com/ui/1.13.1/themes/base/jquery-ui.css">
<script src="https://code.jquery.com/ui/1.13.1/jquery-ui.js"></script>
</head>
Autocomplete Methods
The following methods show how the autocomplete widget can be used. Details on the use of each method available within autocomplete are described below.
close | The close method closes the autocomplete menu. Close is helpful to close the open menu whenever the search method is used, ultimately hiding the suggestions provided. |
destroy | The destroy method is used to remove the autocomplete functionality, and destroy returns the autocomplete element back to its original state. |
disable | The disable method is used to disable autocomplete. |
enable | The enable method enables the autocomplete widget that was previously disabled; it is used to re-enable it. |
instance | The instance method fetches the autocomplete object associated with the element; else, it returns null. |
option | The option method returns the current value of the option name passed as a parameter. |
search | The search method triggers the search event, which takes the current input value and fetches the results against terms in the data source. Passing minLength: 0 and an empty string in the search method retrieves all the words. |
widget | The widget method returns the menu object containing the menu element, created during autocomplete initialization and reused for each search. |
Autocomplete Events
Below we show the jQuery autocomplete events that are triggered by various user and widget actions.
change | The change event is triggered when the value changes. |
close | The close event is triggered when the suggestion menu is closed. |
create | The create event is triggered when the autocomplete is created. |
focus | The focus event is triggered whenever the suggestion is focused. Focusing suggestions’ default behavior is replacing the input value with the focused item. |
open | The open event is triggered whenever the autocomplete suggestions are opened or updated. |
response | The response event is triggered whenever an autocomplete search result is returned, even if there are no results. The response event can transform data before it is updated in the suggestion menu. |
search | The search event is triggered after the delay, and minLength options are met. The search event can be stopped to block a search request to the data source, and no items will be updated in the menu. |
select | The select event is triggered whenever a user selects a value within the suggestion menu and then updates the text field’s value. You can cancel the select event to prevent the text field value from updating. |
Usage and Example
To implement the autocomplete widget, preparing a simple page with a few basic HTML elements is necessary. To start, we must create an <div> element with the class name “example-ui”. Next, we define the <input> element with a <label> and add the “driver” ID to both.
HTML Section:
<div class="example-ui">
<label for="drivers">F1 Drivers: </label>
<input id="drivers">
</div>
Next, we will prepare a list containing the names of F1 drivers in JavaScript as an array.
var availableTags = [
"driver name one",
"driver name two"
];
We will define the F1 drivers that will appear in the text box in the script and use the name as the data source of autocomplete.
$( "#drivers" ).autocomplete({
source: availableTags
});
We’ll merge these two JavaScript pieces and place them into an anonymous function as below. We have added nine names to the array, and you can add additional terms to make the suggestions more dynamic.
Full Script:
<script>
$( function() {
var availableTags = [
"Max Verstappen",
"Daniel Ricciardo",
"Lando Norris",
"Sebastian Vettel",
"Charles Leclerc",
"Lance Stroll",
"Kevin Magnussen",
"Yuki Tsunoda",
"Alexander Albon",
];
$( "#drivers" ).autocomplete({
source: availableTags
});
} );
</script>
When we bring the included header scripts, the HTML elements, and JavaScript sections together, the auto-complete feature is added to the data entry field. In the code example below, the suggestions are taken from the name array and displayed under the text box when the user starts typing in the data entry field.
Full Code:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>F1 Drivers jQuery UI Autocomplete Example</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.13.1/themes/base/jquery-ui.css">
<link rel="stylesheet" href="/resources/demos/style.css">
<script src="https://code.jquery.com/jquery-3.6.0.js"></script>
<script src="https://code.jquery.com/ui/1.13.1/jquery-ui.js"></script>
<script>
$( function() {
var availableTags = [
"Max Verstappen",
"Daniel Ricciardo",
"Lando Norris",
"Sebastian Vettel",
"Nicholas Latifi",
"Pierre Gasly",
"Sergio Perez",
"Fernando Alonso",
"Charles Leclerc",
"Lance Stroll",
"Kevin Magnussen",
"Yuki Tsunoda",
"Alexander Albon",
"Guan Yu Zhou",
"Esteban Ocon",
"Lewis Hamilton",
"Mick Schumacher",
"Carlos Sainz Jr.",
"George Russell",
"Valtteri Bottas"
];
$( "#drivers" ).autocomplete({
source: availableTags
});
} );
</script>
</head>
<body>
<div class="example-ui">
<label for="drivers">F1 Drivers: </label>
<input id="drivers">
</div>
</body>
</html>
Output:
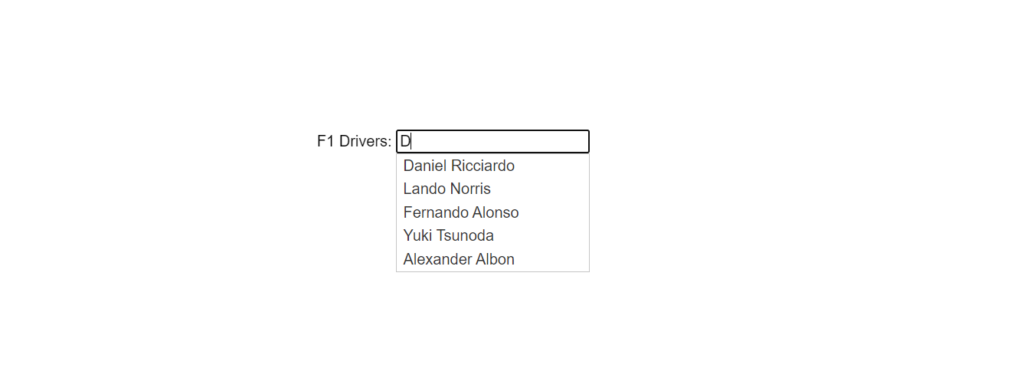
Final Words
The example above is one way the autocomplete jQuery library can be used. You can create different designs using CSS and some of the methods and events within autocomplete. You can expand the features of your web page with advanced autocomplete examples such as combo box, multiple values, remote data source, and accent folding. There is also the possibility of using autocomplete with a remote database source for larger projects. That’s all for today. See you in the following articles.
Recommended Articles
- How To Handle Form Data With jQuery
- Using jQuery To Manipulate Classes and IDs
- How To Add and Remove an Active Class With jQuery
- How To Change the Background Color on Hover With jQuery
- How to Create a jQuery Ajax Contact Form in PHP