This article is an introduction with code, to JavaScript APIs such as third-party APIs, browser APIs like the user history and storage, and the fetch API. Included are code samples to help you understand.
Table of Contents
What Are APIs
APIs are a standard utility across the technology field. In programming, API stands for Application Programming Interface, they extend the functionality of a program, website, or server. Typically they connect to additional software for the retrieve or submission of data. For example, a server may fetch the weather from an external service and then display it on a mobile device’s screen, such as in the case of Android’s home screen, its weather widget, or weather apps. Below is a picture where the outside temperature is displayed under the time. The Weather Channel app fetches the temperature from a remote API.
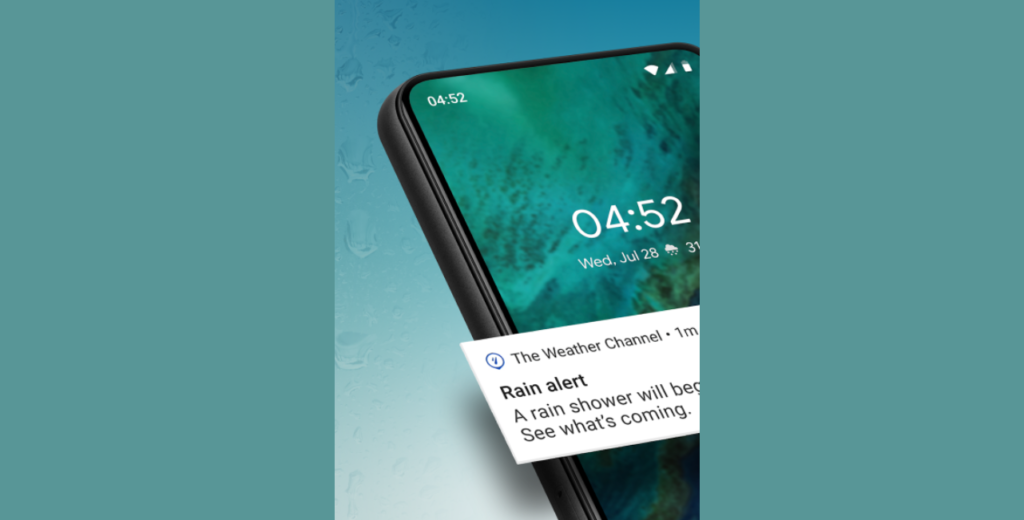
Third-party APIs
What are third-party APIs? Third-party APIs are APIs provided by external software. They allow you to send and receive data to other software or perform actions. Examples of third-party APIs are SMS, email, and cloud server API services. To access these services, you have to understand the APIs are not built into the browser but are accessed by contacting another server or software. Contacting is done through the web or locally programmatically. More examples include Google APIs, Facebook APIs, Twitter APIs, and YouTube APIs.
Browser APIs
What are browser APIs? Browser APIs are APIs used to support complex operations and help ease access to data for websites and web apps. The APIs are either an extension of JavaScript or a feature built into the browser. For example, chrome supports a variety of APIs that are available for consumption.
- audio
- application cache
- canvas
- geolocation
- notifications
- video
- web database
- history
- storage
Web History API
What is the Web History API? The Web History API is a JavaScript extension that provides access to browse history objects, visited pages, and navigation. The following methods are popular to access URLs visited by users.
- The history.back() method
- This method loads the previous URL on the windows. history list.
- This is the same as a back arrow in HTML.
- The history.forward() Method.
- This method loads the next page in the windows. history list.
- The history.go() method
- This method loads a specific URL in the window’s history list.
// Go back in history.
history.back();
// Go forward in history
history.forward();
//Use a number parameter to specify how many pages backwards or forward in history
history.go();
Web Storage API
What is the Web Storage API? The Web Storage API provides a method allowing you to store key and value pairs in the browser with a get and set function using JavaScript. Below, we’ve provided the basic syntax for storing and retrieving data in the browser with the Web Storage API.
Note: The Web Storage API and its syntax are supported by all major browsers.
The localStorage object.
- This syntax provides local storage for a particular website.
- The syntax allows you to store, read, add, modify and delete data for the domain.
- The stored data stays even when the browser is closed.
Example
// Using the local storage object
localStorage.setItem("car","Ford");
localStorage.getItem("car");
The sessionStorage object.
- This system works as the localStorage only in that it stores data for only one session.
- The data deletes when the browser is closed.
Example
// Using the session storage object
sessionStorage.setItem("fruit","Apple");
sessionStorage.getItem("fruit");
1. The setItem() method.
The setItem() method stores data items in the storage and it takes a name and value as parameters.
2. The getItem() method.
The getItem() method retrieves data items from the storage but only the name as the parameter.
Web Workers API
What is the Web Worker API? The Web Worker API is used to create JavaScript that runs independently in the background and doesn’t affect the performance of the page. Workers enable users to handle complex executions in the background without interfering with the running of the main UI thread. Essentially the web workers allow multithreading since JavaScript is purely single-threaded, that is, it doesn’t allow the running of more than one script at the same time.
The main thread and web workers communicate through the postMessage() method and onmessage event handler.
We’ve written a useful demonstrative article on how to create a Web Worker using the Web Worker API. The article contains a web worker which counts and updates an element on the web page. Direct Link →
Web Geolocation API
What is the Web Geolocation API? The Web Geolocation API enables a script to locate a user’s position. There are two major location APIS, the JavaScript Geolocation API, and the Google Geolocation API, which both can be used to get the geographical position of the user.
Fetch API
What is the Fetch API? The Fetch API provides a method to get resources from the web or local network, similar to the less powerful XMLHttpRequest
feature.
Below is the basic syntax of fetching a file and displaying the content using the fetch method. A URL is used as a parameter and then the received data is converted to text and displayed.
fetch(url)
.then(x => x.text())
.then(y => myDisplay(y));
Another way of using the Fetch API is based on async and await. Using async is a little more complex than the single method but gives you the benefit of asynchronous, promise-based behavior.
async function getText(url) {
let x = await fetch(url);
let y = await x.text();
myDisplay(y);
}
Example of async fetch
Now let’s look at how to implement a Javascript Fetch API using the async() and await() functions. In this example, we will be fetching data from a mock API I already created and then displaying the details in HTML format.
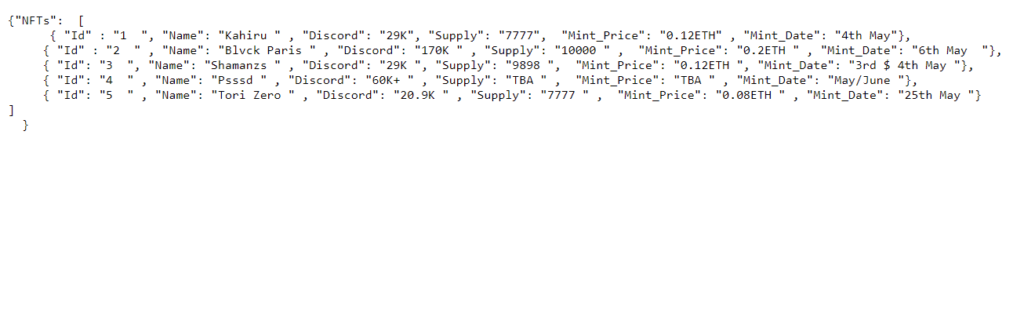
- Copy the URL of your API
https://appcode.free.beeceptor.com/my/api/Json/NFTs
- Define an async() function
async function getDataFromAPI(url) {
// Function logic
}
- Set a response variable and equate it to the await() function. Inside this function, pass the API URL.
let x = await fetch(url);
- Store the data in JSON format using the response.json() function
let y = x.json();
- Log the data on the console
console.log(y);
- Define the table data, in this case, we used a for loop
length = data.NFTs.length;
for (i = 0; i < length; i++) {
temp += "<tr>";
temp += "<td>" + data.NFTs[i].Id + "</td>";
temp += "<td>" + data.NFTs[i].Name + "</td>";
temp += "<td>" + data.NFTs[i].Supply + "</td>";
temp += "<td>" + data.NFTs[i].Mint_Price + "</td>";
temp += "<td>" + data.NFTs[i].Mint_Date + "</td>";
}
- Set the innerHTML as a temp/tab variable, in our case a temp variable.
document.getElementById("data").innerHTML = temp;
Below is the output when you test that code
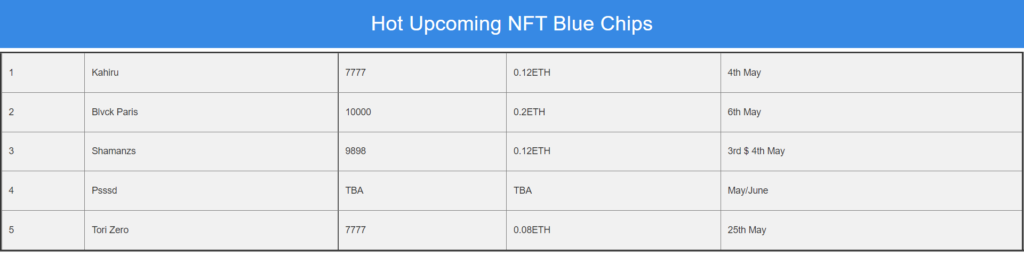
You can view and test the entire fetch project on CodePen via this link.
Recommended Articles
- Using the JavaScript Web Workers API
- What Are JavaScript Arrays, and How To Use Them?
- JavaScript Event Listeners for Every Newbie Developer
- Introduction to the Fancy JavaScript Request
- Encapsulating CSS with JavaScript and Shadow DOM