This article demonstrates how to use the JavaScript Web Workers API with code examples. Included are methods and live examples to get started.
- How Do You Create a Web Worker
- Web Worker JavaScript File
- Worker onmessage Event Listener
- Terminating or Stopping a Web Worker
- Web Worker Example
How Do You Create a Web Worker
- Create a web worker in an external JavaScript. Then store the script in the “workers.js” file
- Inside your HTML body, define a web worker object/variable.
- On your HTML file, check if the web worker already exists using the following code
if (typeof myWorker == "undefined") {
myWorker = new Worker("worker.js");
}
If the worker doesn’t exist, the above code creates a new web worker object and runs the ‘worker.js’ file.
Web Worker JavaScript File
- Create the worker.js file with some functions and actions
- Use the postMessage() method to send data to an event listener in the main thread.
The Web Worker script below is an example where a timed counter is posted back to the web page every 500 milliseconds.
// Defining our worker in a separate script, runs in the background, post results to main thread (UI)
let i = 0;
function timedCount() {
i++;
postMessage(i);
setTimeout("timedCount()", 500);
}
timedCount();
Worker onmessage Event Listener
- Call the web worker to your HTML page using the onmessage event listener
- When the web worker posts a message/execution result, the code within the event listener is executed. The data from the web worker is stored in event.data.
- Have the event listener perform an action when an event is found.
Here is an example of an event listener function. The element with the ID of “result” has its inner HTML set to the event data whenever the worker uses the
myWorker.onmessage = function (event) {
document.getElementById("result").innerHTML = event.data
};
Terminating or Stopping a Web Worker
- Terminate the web worker using the terminate() method:
- The terminate method destroys the web worker object.
myWorker.terminate();
Why should I terminate or stop a web worker?
We end the web worker because once a web worker object or variable has been created, it will continue to run and send events. Also, the event listener will continue to hold memory space even after the external worker script is finished executing. Therefore to free browser/computer processor and memory resources, you should terminate workers for best practice. Direct Link →
- It’s optional to set the worker variable as undefined to enable its reuse later.
myWorker = undefined;
- You can create a custom stop function for web workers.
function stopWorker() {
myWorker.terminate();
myWorker = undefined;
}
Web Worker Example
You may view and test an example of a web worker counter on codesandbox.io
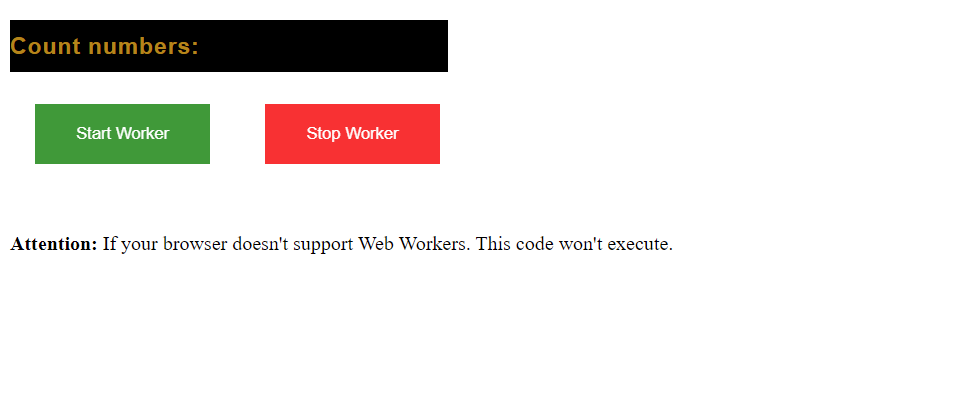
Recommended Articles
- What Are JavaScript Arrays, and How To Use Them?
- JavaScript Event Listeners for Every Newbie Developer
- JavaScript Fundamentals – A Dinosaurs Cheat Sheet
- Introduction to the Fancy JavaScript Request
- Encapsulating CSS with JavaScript and Shadow DOM