This article demonstrates how to use the CSS translate function on elements with CSS transform. Included are code, definitions, and examples.
The CSS translate function defines a 2D translation across the vector [tx, ty]
. Translate repositions HTML elements using both the tx
and ty
vectors in the horizontal and/or vertical directions. Such as up and down, or left and right.
The translate()
function is a <translate-function>
data type.
Since the translation of the element is characterized by a two-dimensional vector coordinate, the element’s position is defined by the value of each coordinate. By changing the value of either the tx
or ty
coordinates, you can change the position of an element from its starting position.
Syntax
transform: translate(tx, ty);
Parameters
tx
The tx value of translate() is either a number or a percentage. The tx
coordinate represents the x-axis of an element. A positive value will move the element up, while a negative value will move the HTML down. This first coordinate is required to use translate.
ty
The ty value of translate is either a number or percentage. The ty
coordinate represents the y-axis of an element. A positive value will move the HTML element right, while a negative value will move the element left. This parameter is optional and by default the value is zero if omitted.
Examples
The examples below use numbers and percentage values for the parameters of the translate function.
/* Single <length-percentage> values */
transform: translate(300px);
transform: translate(60%);
transform: translate(25%);
/* Double <length-percentage> values */
transform: translate(50px, 100px);
transform: translate(100px, 50%);
transform: translate(10%, 100px);
transform: translate(50%, 75%);
/* Negative values */
transform: translate(-16%);
transform: translate(-6px, -30px);
Usage
You can apply the translate function to any element or image by using CSS selectors.
#element-id {
transform: translate(10%);
}
.element-class {
transform: translate(25px, 25px);
}
img {
transform: translate(-50%, -50%);
}
Try It
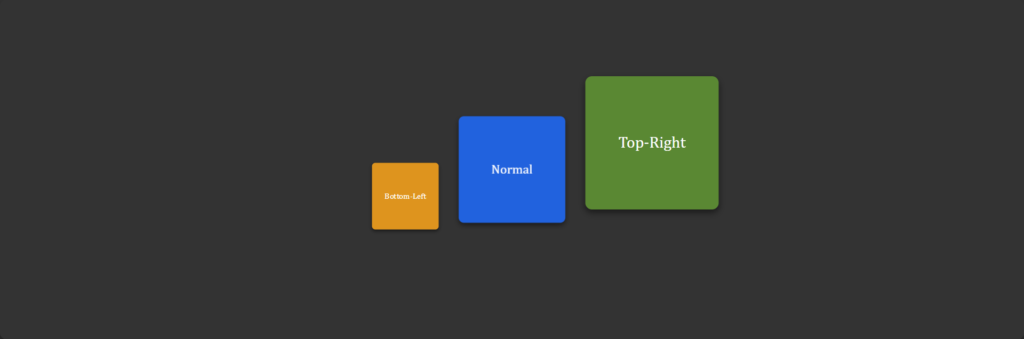
HTML
<div class="container">
<div class="perspective">
<p>Normal</p>
</div>
<div class="bottom-left">
<p>Bottom-Left</p>
</div>
<div class="top-right">
<p>Top-Right</p>
</div>
</div>
CSS
body,
html {
height: 100%;
margin: 0;
background: #333;
font-size: 24px;
}
body {
color: white;
}
div {
width: 200px;
height: 200px;
display: inline-block;
background-color: #ffa500db;
border-radius: 10px;
box-shadow: 0 5px 10px rgb(0 0 0 / 50%);
}
.perspective {
background-color: #1560e0;
/* Includes a perspective to create a 3D space */
transform: perspective(400px) translateZ(-100px);
position: absolute;
z-index: 1;
}
p {
font-family: cambria;
line-height: 150px;
}
.container {
width: 100%;
background: transparent;
text-align: center;
-webkit-box-align: center !important;
-ms-flex-align: center !important;
align-items: center !important;
-webkit-box-pack: center !important;
-ms-flex-pack: center !important;
justify-content: center !important;
display: -webkit-box !important;
display: -ms-flexbox !important;
display: flex !important;
height: 100%;
position: relative;
}
.scaled-translated {
/* Includes a perspective to create a 3D space */
transform: perspective(400px) scaleZ(2.5) translateZ(-100px);
background-color: #56882d;
}
.bottom-left {
position: absolute;
transform: translate(-160px, 40px) scale(0.5);
}
.top-right {
position: absolute;
transform: translate(210px, -40px);
background-color: #56882d;
}
Single X-axis Translation
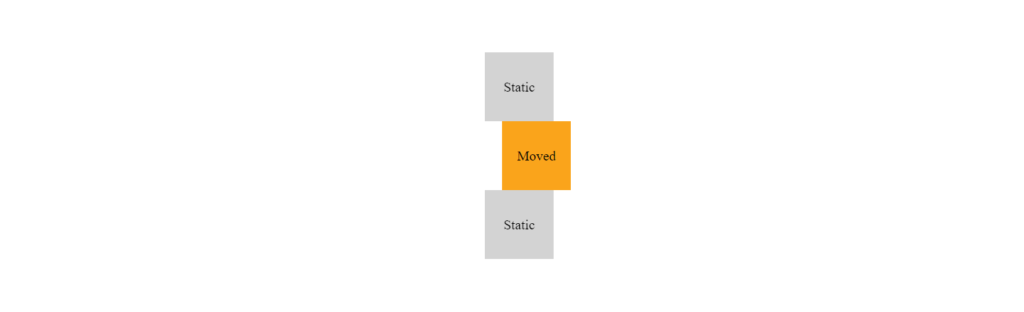
HTML
<div class="box">
<div>Static</div>
<div class="moved">Moved</div>
<div>Static</div>
</div>
CSS
html,
body {
height: 100%;
margin: 0;
-webkit-box-align: center !important;
-ms-flex-align: center !important;
align-items: center !important;
-webkit-box-pack: center !important;
-ms-flex-pack: center !important;
justify-content: center !important;
display: -webkit-box !important;
display: -ms-flexbox !important;
display: flex !important;
}
.box {
height: 240px;
}
.box div {
line-height: 80px;
text-align: center;
width: 80px;
height: 80px;
background: lightgray;
}
.moved {
/* Equal to: translateX(25%) or translate(25%, 0) */
transform: translate(25%);
background: orange !important;
}
Both X-Axis and Y-Axis Translation
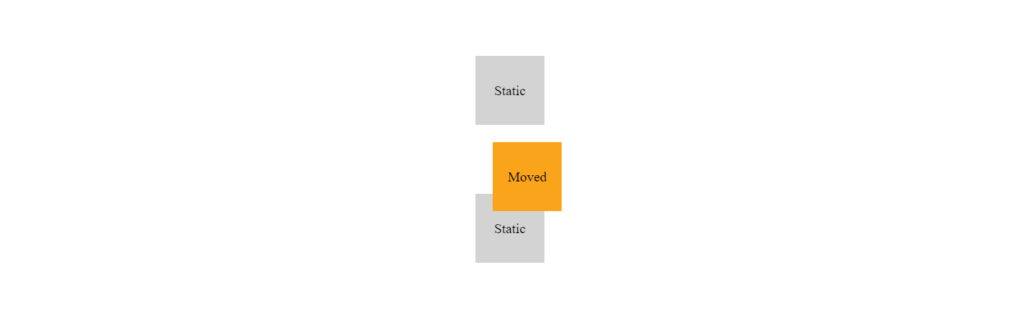
HTML
<div class="box">
<div>Static</div>
<div class="moved">Moved</div>
<div>Static</div>
</div>
CSS
html,
body {
height: 100%;
margin: 0;
-webkit-box-align: center !important;
-ms-flex-align: center !important;
align-items: center !important;
-webkit-box-pack: center !important;
-ms-flex-pack: center !important;
justify-content: center !important;
display: -webkit-box !important;
display: -ms-flexbox !important;
display: flex !important;
}
.box {
height: 240px;
}
.box div {
line-height: 80px;
text-align: center;
width: 80px;
height: 80px;
background: lightgray;
}
.moved {
transform: translate(25%, 25%);
background: orange !important;
}
Specifications
W3C CSS Transforms Module Level 1
#two-d-transform-functions