This article demonstrates how to add or remove a CSS class using jQuery methods to manipulate a class on an HTML element when clicked.
Manipulating Classes With jQuery Methods
Sometimes it’s necessary to switch CSS classes when an action is performed on a website. We will explore two methods to add & remove or toggle classes using jQuery functions. These methods include the addClass()
, removeClass()
, toggleClass()
functions.
Here are three frequently asked questions:
- How Do You Remove an Active Class?
The removeClass()
jQuery function removes one or more CSS class names from the target elements. Additionally, removeClass()
will remove all class names from the target element if no parameter is supplied.
- How Do You Add an Active Class?
The addClass()
jQuery function adds one or more classes to target elements. This method only adds one or more classes to the class attribute and will not remove existing CSS classes. A useful feature is you can supply addClass with more than one class in the parameter by separating the names with spaces.
- How Do You Toggle an Active Class?
The toggleClass()
function checks each target element against the supplied class name or names list parameter and toggles between adding and removing classes from the elements. This method creates a toggle effect because the class names are added if missing and removed if they already exist.
Creating the Walkthrough Example
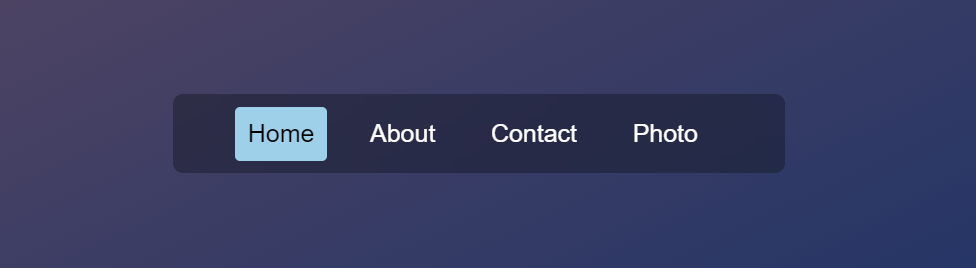
The first task we need to perform is creating an HTML example so we can use jQuery methods to manipulate CSS classes. Down below, we use HTML to construct an unordered list menu. The menu consists of four list elements. Additionally, the first menu includes a class called .active
. This class name, .active
, will be the class that is changed using jQuery methods.
HTML
<div class="menu-container">
<ul class="menu">
<li class="active">Home</li>
<li>About</li>
<li>Contact</li>
<li>Photo</li>
</ul>
</div>
CSS styles below give the menu visibility and structure for this demonstration. We use a CSS gradient on an HTML and Body element while using CSS flexbox to center a menu vertically and horizontally. As you see below, we define some styles for the .active
class. When added as a name to the class attribute on an HTML element, the .active
class sets the CSS text color to black and the CSS background to #9bd0eb
and text to a color of black.
CSS
.menu {
list-style: none;
}
.menu li {
font-size: 20px;
margin: 10px;
padding: 10px;
cursor: pointer;
display: inline-block;
border-radius: 4px;
}
.menu {
width: 450px;
margin: 0 auto;
background: rgba(16 18 27 / 40%);
border-radius: 8px;
backdrop-filter: blur(20px);
}
.menu-container {
margin: 0;
padding: 0;
height: 100%;
-webkit-box-align: center !important;
-ms-flex-align: center !important;
align-items: center !important;
-webkit-box-pack: center !important;
-ms-flex-pack: center !important;
justify-content: center !important;
display: -webkit-box !important;
display: -ms-flexbox !important;
display: flex !important;
}
.active {
background: #9bd0eb;
color: black;
}
Toggle Using removeClass() & addClass()
This method of toggling classes on an element consists of mixing the removeClass()
and a addClass()
method immediately after. The example below removes any active classes matching a list element that currently has an .active
class name. Then the .active
class is applied to the element when it is clicked.
$('.menu').on('click', 'li', function() {
$('.menu li.active').removeClass('active');
$(this).addClass('active');
});
Edit this demonstration on codepen.io
Using toggleClass() To Toggle a CSS Class
A second method is similar to the first and uses removeClass()
to clear all class names matching .active
. Then instead of using addClass()
we use toggleClass()
to toggle the active class onto the element clicked since the element clicked does not have .active
.
$('.menu').on('click', 'li', function() {
$('.menu li.active').removeClass('active');
$(this).toggleClass('active');
});
jQuery
We use jQuery in the few examples above. jQuery is a library framework of JavaScript that provides easy-to-use methods. In addition, jQuery makes it easy to select elements by selector reference. Selecting elements is essential because it allows us to run functions on any element.
jQuery can easily be installed by including the script below in the header of each page.
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
</head>
Recommended Articles
- How To Change Text Color on Hover With jQuery
- How To Handle Form Data With jQuery
- How To Change the Background Color on Hover With jQuery
- How to Create a jQuery Ajax Contact Form in PHP
- How To Use Autocomplete for jQuery With a Text Array Data Source
- Using jQuery To Manipulate Classes and IDs