This article demonstrates how to use the web geolocation API. Methods, properties, examples, demo, and geolocation API code are included.
What Is the Web Geolocation API
The geolocation API allows users to give their location data to web applications, pages, and sites. The API provides access to a location object containing the user’s latitude, longitude, altitude, heading, timestamp, and speed. Addionally you can retrieve the location in intervals to check if the user’s position has changed. For privacy reasons, the user must accept permission to share the location data with a website before a site can use it.
Table of Contents
- Using the Geolocation API
- Handling Errors and Rejections
- Displaying the Result in a Map
- Location-specific Information
- Location Properties and Methods
Using the Geolocation API
The getCurrentPosition() method is used to return the user’s position. Here is the syntax for using the method.
navigator.geolocation.getCurrentPosition(success, error, options);
As you see above, there are three parameters to the getCurrentPosition() method. We’ll discuss each.
- success – The getCurrentPosition success parameter is a callback function triggered whenever the location has been fetched.
- error – The getCurrentPosition error parameter is a callback function for errors thrown when receiving the location.
- options – The getCurrentPosition options parameters are for passing options.
Note: The error and option parameters are optional, while success is required for the method to work.
Let’s create an example using the new method we discussed. The javascript example below returns the latitude and longitude of any user’s position:
const x = document.getElementById("demo");
function getLocation() {
try {
navigator.geolocation.getCurrentPosition(showPosition, showError);
} catch {
x.innerHTML = err;
}
}
function showPosition(position) {
x.innerHTML =
"Latitude: " +
position.coords.latitude +
"<br>Longitude: " +
position.coords.longitude;
}
Let’s walk through the code and explain the different sections.
- First, the code checks if geolocation is supported using a try and catch function.
try {
navigator.geolocation.getCurrentPosition(showPosition, showError);
}
- If supported, it runs the getCurrentPosition() method. If the geolocation is not supported, it displays a message to the user using the innerHTML on the element attached to the x variable.
} catch {
x.innerHTML = err;
}
- If the getCurrentPosition() method is successful, it passes a coordinates object to the showPosition() method because the showPosition() was passed as the success parameter. The showPosition() function outputs the latitude and longitude as the innerHTML of the x var element.
function showPosition(position) {
x.innerHTML =
"Latitude: " +
position.coords.latitude +
"<br>Longitude: " +
position.coords.longitude;
}
The example above is a basic geolocation script with no error handling. You can view the complete code on CodePen here.
Handling Errors and Rejections
The second parameter of the getCurrentPosition() method is used to handle errors. It specifies a function to run if it fails to get the user’s location.
navigator.geolocation.getCurrentPosition(showPosition, showError);
Here is a function to handle the various errors fetching the user’s location. We’ve added a case switch to catch the permission denied, position unavailable, timeout, and unknown errors.
function showError(error) {
switch (error.code) {
case error.PERMISSION_DENIED:
x.innerHTML = "User denied the request for Geolocation.";
break;
case error.POSITION_UNAVAILABLE:
x.innerHTML = "Location information is unavailable.";
break;
case error.TIMEOUT:
x.innerHTML = "The request to get user location timed out.";
break;
case error.UNKNOWN_ERROR:
x.innerHTML = "An unknown error occurred.";
break;
}
}
Displaying the Result in a Map
Now it’s time to try creating a more advanced location output using maps. To display the result in a map, you need access to a map service, like Google Maps, using the Maps JavaScript API
The example below shows the returned latitude and longitude from the success function to render the location in a Google Map (using a static image).
Note: You will need to provide your Maps API key to get the example to work. The key can be updated in the query key parameter, &key=YOUR_KEY, in the below code.
function showPosition(position) {
let latlon = position.coords.latitude + "," + position.coords.longitude;
let img_url = "https://maps.googleapis.com/maps/api/staticmap?center=
"+latlon+"&zoom=14&size=400x300&sensor=false&key=YOUR_KEY";
document.getElementById("mapholder").innerHTML = "<img src='"+img_url+"'>";
}
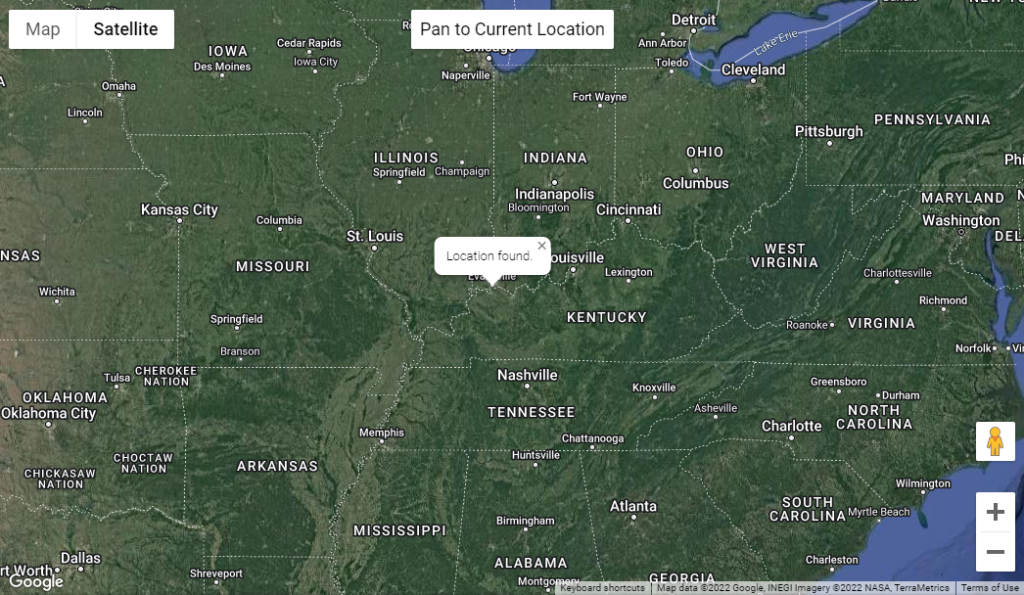
You can see the example of a map display of your location here on codesandbox.io
Location-specific Information
In the example above, we demonstrated how to show a user’s position on a map. Geolocation is also very useful for fetching location-specific information in creating features like:
- Up-to-date local information
- Showing points of interest near the user
- Turn-by-turn navigation (GPS)
Location Properties and Methods
Below are the geolocation properties and methods.
Geolocation Object Properties
coordinates | The coordinates property returns the altitude and position of the device. |
position | The position property returns the set of coordinates and the device’s timestamp. |
positionError | The positionError property returns the error message when trying to retrieve the location. |
positionOptions | The positionOptions property is the enableHighAccuracy , timeout, and maximumAge options which are passed as an object parameter through the Geolocation.getCurrentPosition() and Geolocation.watchPosition() methods. |
Geolocation Object Methods
clearWatch() | The clearWatch method unregisters the location and error event handler set up by the watchPosition method. |
getCurrentPosition() | The getCurrentPosition method returns the current position of the device. |
watchPosition() | The watchPosition method creates an event handler that triggers a callback method every time the event is triggered. An option object can be passed a third parameter to control the location cache, frequency, and accuracy. |
Geolocation Coordinates Properties
Property | Returns |
coordinates.latitude | The coordinates latitude property returns the latitude as a decimal number. |
coordinates.longitude | The coordinates longitude property returns the longitude as a decimal number. |
coordinates.altitude | The coordinates altitude property is provided as meters relative to the mean sea level. |
coordinates.accuracy | The coordinates accuracy property returns the accuracy of the coordinates (latitude and longitude properties) in meters. |
coordinates.altitudeAccuracy | The coordinates altitudeAccuracy property returns the accuracy of the altitude property in meters. |
coordinates.heading | The coordinates heading property returns the heading as degrees clockwise from North. |
coordinates.speed | The coordinates speed property returns the device’s speed in meters per second. |
Geolocation Position Properties
position.coords | The position coords property returns an object containing the latitude and longitude. |
position.timestamp | The position timestamp property returns the date and time when a location for the device is captured. |
Recommended Articles
- A Brief Intro to JavaScript APIs
- Using the JavaScript Web Workers API
- Quickly Search and Filter a List With JavaScript
- What Are JavaScript Arrays, and How To Use Them?