This tutorial demonstrates various methods including CSS in react components such as Sass, inline CSS, external CSS, constants, and variables.
HTML is used to structure a web page and its content in web development, while JavaScript is used to make the web pages interactive and dynamic. But modern websites are not only HTML and JavaScript. How the website looks is as important as structuring it or making it dynamic. In fact, the website presentation is more important because no one wants to visit an ugly and unattractive website.
In the modern web, you cannot have a website without CSS. Today the websites are attractive, they have animations, and most importantly, they run on every device. All this is because of CSS.
For a React developer, it is essential to learn how to use CSS with React. There are several different ways of using CSS with React. This article will discuss the most commonly used methods, including CSS with React such as inline CSS, external CSS, and variables. Moreover, we will also discuss what Sass is and how it is used with React.
React and CSS
React is a JavaScript library developed and maintained by Facebook. It is used to create dynamic and interactive user interfaces, and it is one of the most popular web development libraries in the world. It uses JSX, a JavaScript extension that enables the developers to write HTML and CSS in JavaScript.
There are several ways of using CSS in React. Some of the commonly used methods are listed below.
⦁ Standard CSS
⦁ Inline CSS
⦁ Sass
⦁ Radium
⦁ JSS
⦁ styled-components
Every React developer should be familiar with the first two ways mentioned above. Using standard CSS and inline CSS requires only basic CSS knowledge. Apart from them, Sass is another popular way to use CSS with React.
Standard CSS
Standard CSS is the most commonly used way to implement CSS in React applications. In this method, a CSS file is created, and then it is imported into the component.
Observe the following component.
const App = () => {
return (
<div>
<h2>Hello World!</h2>
<p>My name is Johny. I am from Brampton, Canada.</p>
</div>
);
};
export default App;
As of now, no CSS is applied to any of the HTML elements in the App component.
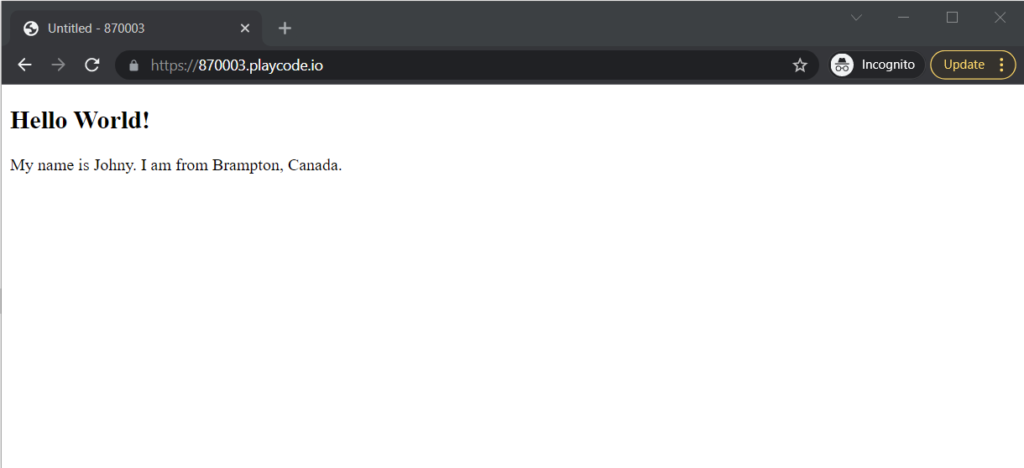
Let’s create a CSS file and name it “styles.css”.
.app {
padding: 20px;
font-family: 'Lato', 'Lucida Grande', 'Lucida Sans Unicode', Tahoma, Sans-Serif;
}
.heading {
color: #6b7790;
}
.para {
color: white;
font-size: 20px;
padding: 4px;
}
body, html {
margin: 0;
height: 100%;
background: linear-gradient(60deg, rgb(39 28 86) 0%, rgb(0 85 96) 100%);
}
This file creates three classes in a standard CSS way using the dot (.) notation. Then, several properties with values are defined in each class, again like standard CSS.
To use this CSS in the App component, we first have to import it and then use the classes using the “className” attribute, which is the equivalent of “class” in HTML.
className="app"
Let’s add the three classes to the React App component.
import "./styles.css";
const App = () => {
return (
<div className="app">
<h2 className="heading">Hello World!</h2>
<p className="para">My name is Johny. I am from Brampton, Canada.</p>
</div>
);
};
export default App;
You can notice how applying classes in React is the same as applying classes in HTML. Let’s check the output.
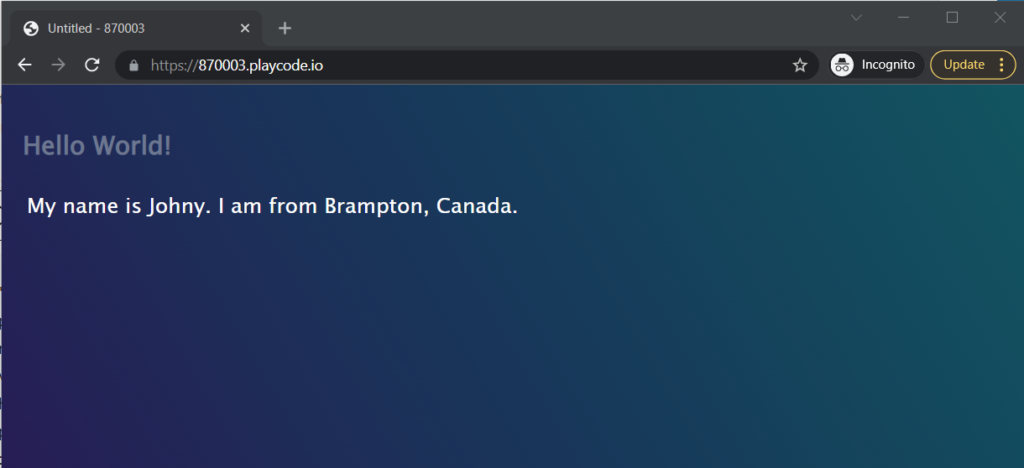
Yes, the CSS is applied! We’ve successfully used the classes from the imported styles.css file.
Inline CSS
Inline CSS is another commonly used way in React, though it is recommended to use it as few times as possible. CSS is applied to a particular component in the JavaScript file only in this method. The style attribute is used, and an object with CSS properties is provided as its value.
style={{ color: "green" }}
^ ^
| |-- Style object includes CSS properties wrapped in double brackets
|
|-- Style attribute with an equal sign
Observe the style attribute of the h2 element.
const App = () => {
return (
<div>
<h2 style={{ color: "green" }}
>Hello World!</h2>
<p>My name is Johny. I am from Brampton, Canada.</p>
</div>
);
};
export default App;
This inline CSS will apply a “green” color to the h2 element.
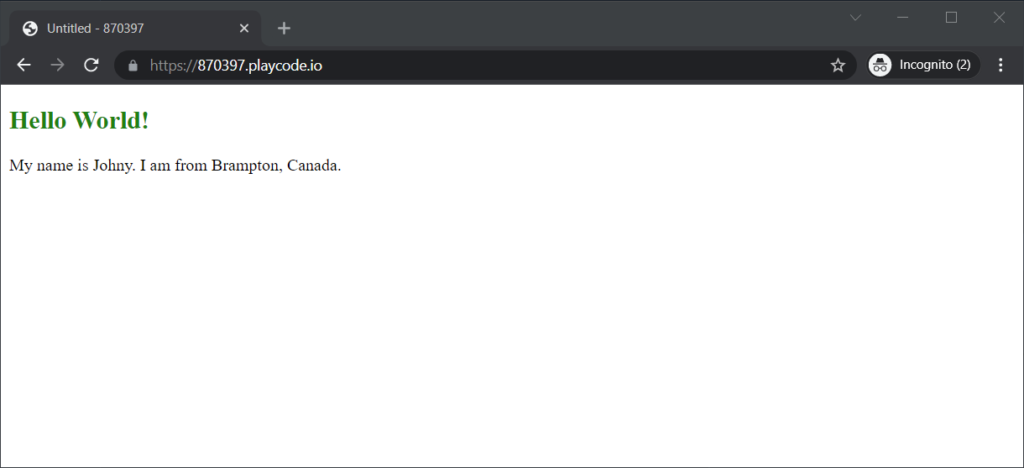
Now, here is a more advanced example. Let’s apply the same CSS to the div, which uses the inline CSS way also.
const App = () => {
return (
<div style={{ backgroundColor: "darkblue", textAlign: "center" }}>
<h2 style={{ color: "green" }}>Hello World!</h2>
<p>My name is Johny. I am from Brampton, Canada.</p>
</div>
);
};
export default App;
First, the multiple properties are separated using a comma, and second, the properties that had a hyphen in their names are written in camel-case. For example, “background-color” became “backgroundColor” and “text-align” became “textAlign”.
style={{ backgroundColor: "darkblue", textAlign: "center" }}
Similarly, let’s apply new styles to the <div> and <h2> elements and add CSS to the <p> element.
const App = () => {
return (
<div style={{ padding: "20px", fontFamily: "Sans-Serif", background: "#18222d" }}>
<h2 style={{ color: "#a3a3a3" }}>Hello World!</h2>
<p style={{ color: "white" }}>My name is Johny. I am from Brampton, Canada.</p>
</div>
);
};
export default App;
With inline CSS, there is another difference. If the value has a unit, for example, px or rem, it should be provided as a string, as we did for the <p> tag.
padding: 20px; /* The pixal unit becomes a string. */ padding: "20px";
Let’s check the output.
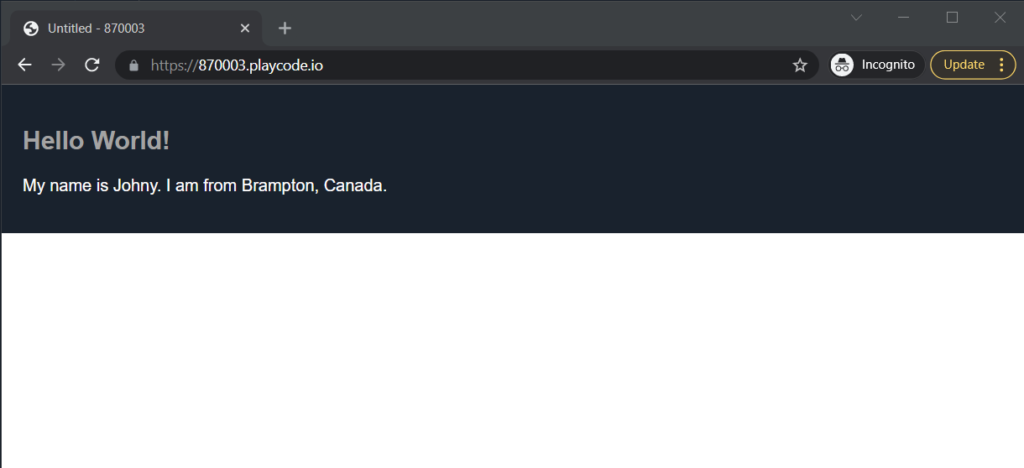
Inlining CSS into react works ideally, but inline CSS is not recommended because it creates a mess in the JSX code. We can create objects to make the inline CSS look better to avoid clutter. Objects can be created using constants and a variable name.
const importantStyles = {
color: "black",
background: "white"
}
The variable object can now be called using brackets and the name of the constant.
<div style={importantStyles}>
Let’s apply real objects to the react App component.
const divStyles = {
background: "linear-gradient(to right, #201d2e, #bdc3c7)",
textAlign: "center",
padding: "20px",
fontFamily: "Sans-Serif"
};
const headingStyles = {
color: "#dfdfdf",
};
const paragraphStyles = {
color: "white",
fontSize: "20px",
padding: "4px",
};
const App = () => {
return (
<div style={divStyles}>
<h2 style={headingStyles}>Hello World!</h2>
<p style={paragraphStyles}>
My name is Johny. I am from Brampton, Canada.
</p>
</div>
);
};
export default App;
Output using the variables objects.
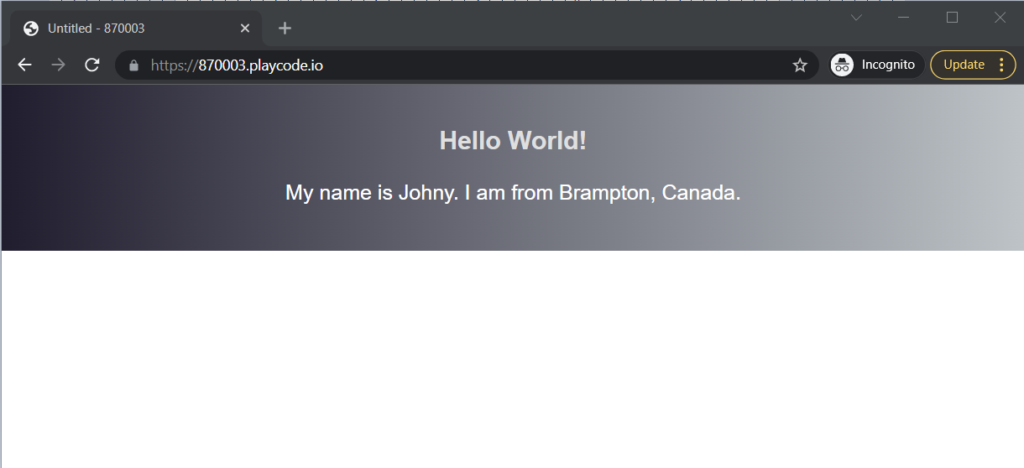
So, this makes the code less messy! But remember, the inline CSS should be used as little as possible.
What is Sass?
CSS is enormous and has so many “things” in it that even a professional, experienced developer struggles while working with it. It is hard to understand CSS, and it is pretty challenging to implement CSS over the entire application. Moreover, CSS has many syntax limitations. To counter these CSS problems, preprocessors have been introduced.
A CSS preprocessor is a layer between stylesheets in your application and the CSS file that is served to the browsers. Sass is one such CSS preprocessor that is widely used with React applications.
Sass stands for Syntactically Awesome Stylesheets, and as the name suggests, it is a kind of syntactic sugar for CSS. So what Sass does is add more features that help the developer while applying CSS. It adds variables, nesting, mixins, inheritance, operators, and more.
For example, we are using a specific color in many places around the CSS in the application. Then the requirement changes, and we need to replace that color with a new color. Now, this will be trouble because we need to go to every CSS file where that color is used to change it. But with Sass, we can create a variable for that color and use it all around the application. When we need to change the color, we can change the variable’s value.
Sass in React
Let’s understand how to use Sass in a React application. The first step is to install the Sass module using NPM or yarn.
npm install node-sass
Then, create a new file in the “src” folder and name it “app.scss”. Now, observe the App component.
const App = () => {
return (
<div>
<h2>Hello World!</h2>
<p>My name is Johny. I am from Brampton, Canada.</p>
</div>
);
};
export default App;
The <div> element has a <h2> element and a <p> element. Suppose we want the color of both these elements to be red. Following is the standard CSS way.
.heading {
color: red
}
.para {
color: red
}
Now, let’s do the Sass way.
In the “app.sass” file, first, we will create a variable that will hold the value of the color, and then we will use the variable for the classes of both elements.
$text-color: #c90000;
.heading {
color: $text-color;
}
.para {
color: $text-color
}
What’s the difference? In the standard CSS way, we have to provide the name of the color wherever it is needed, while in the Sass way, we created a variable and used it everywhere. The most significant benefit is that if we need to change the color, we can change the variable’s value.
Let’s import the Sass file in the “App.js” and check the output.
import "./app.scss";
const App = () => {
return (
<div>
<h2 className="heading">Hello World!</h2>
<p className="para">My name is Johny. I am from Brampton, Canada.</p>
</div>
);
};
export default App;
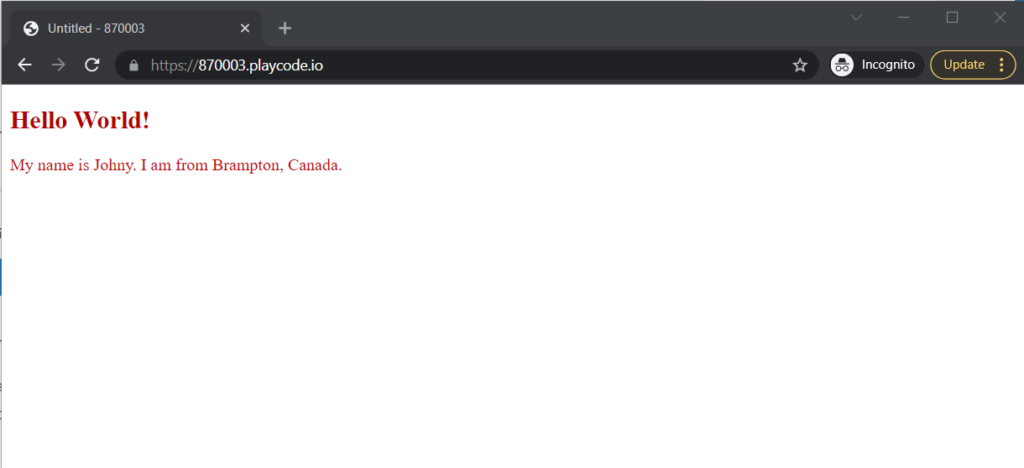
So, Sass is a CSS preprocessor that is syntactic sugar for CSS. It eases the time a developer needs when styling by reducing the amount of work. Above was a basic example of Sass. There are several other features in Sass that can be used with React.
Wrapping it up
Understanding CSS is vital for a frontend web developer. You cannot survive frontend web development without CSS knowledge. The same applies to React developers. The first step is to understand what CSS is and its syntax. Then, learn how to use React using the standard and inline way. Then you can move to advanced stuff such as Sass and styled-components.
Recommended Articles
- Quickly Search and Filter a List With JavaScript
- How To Build a JavaScript Retro Galactic Snake Game
- What Are JavaScript Arrays, and How To Use Them?
- JavaScript Event Listeners for Every Newbie Developer
- Introduction to the Fancy JavaScript Request
- JavaScript Fundamentals – A Dinosaurs Cheat Sheet
- How to use Tilt.js Javascript Library
- Encapsulating CSS with JavaScript and Shadow DOM