AppCode
This is a simple contact form made in PHP with JQuery Ajax. Using Ajax we can avoid using a submit where you don’t want to refresh the page.
This article was first seen and republished from perfectwebtutorials.com
Using jQuery Ajax we can avoid using a submit where you don’t want to refresh the whole page.
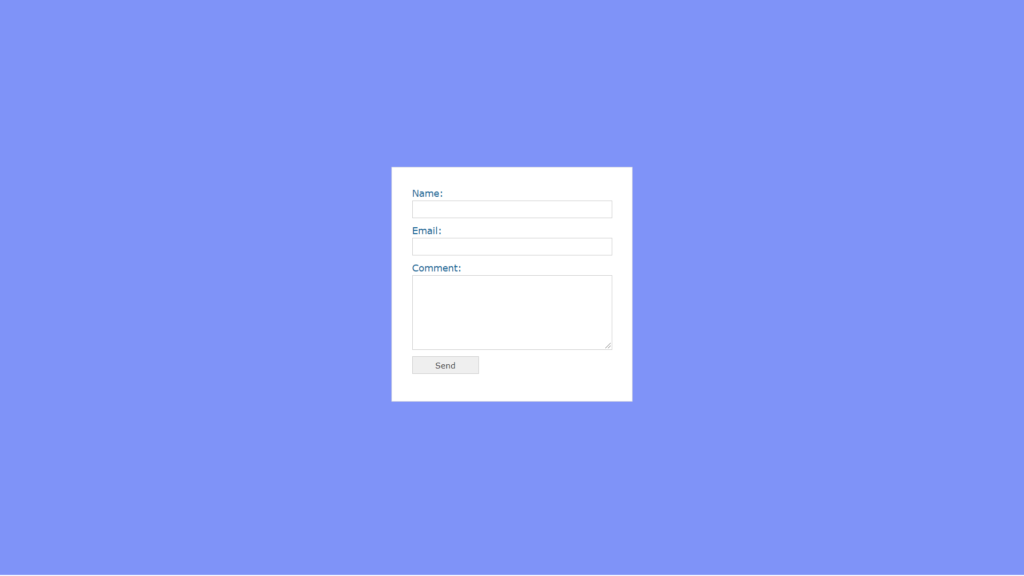
In this tutorial we will use:
- HTML – for designing our form structure. We will use just text fields with one submit button.
- CSS – design our structure styles. Simple CSS, but #error and #loading classes are important
- JQuery – to check our contact form validation before sending to PHP, like if all fields are filled. We can do this in our PHP code too but, if we have a chance to do client-side validation, we should prefer that in case we don’t want to make SERVER-REQUEST.
- PHP code – sending mail.
Let’s Start!
First, create an HTML document and add the following code:
<div id="contactform">
<form action="index.php" method="post">
<label for="name">Name:</label>
<input type="text" name="name" id="name" />
<label for="email">Email:</label>
<input type="text" name="email" id="email" />
<label for="comment">Comment:</label>
<textarea name="comment" id="comment"></textarea>
<input type="submit" value="Send" name="submit" id="submit" />
<img src="loading.gif" id="loading" alt="Wait" />
</form>
</div>
Then our PHP code.
//if we got POST method from our JavaScript Code
<?php
if ($_POST) {
// if all informations are filled
if (($_POST[name]) & ($_POST[email]) & ($_POST[comment])) {
// Your email address
$to = "[email protected]";
// Get name value
$name = $_POST['name'];
// Get email value
$email = $_POST['email'];
// Get comment text
$comment = $_POST['comment'];
// Email tile
// if client name is Robert, title will be "Robert send you an email"
$title = "$name send you an email";
// Email parameters
$mailText .= "From: $namen";
$mailText .= "E-mail: $emailn";
$mailText .= "Comment:n";
$mailText .= $comment;
// Set "From Header parameter"
$headers = 'From:' . $email;
// Send email
$sendMail = mail($to, $title, $mailText, $headers);
// If email is sucessful send
if ($sendMail) {
// Write sucessful message
echo "<strong>$name</strong>, we recived your comment.";
exit;
// If email is NOT sucessful send
} else {
// Write message
echo "<strong>Error</strong>, please try again.";
exit;
}
// If we dont have POST method
} else {
// Write message
echo "<strong>Error</strong>, please try again.";
exit;
}
}
?>
Then we create our JavaScript code.
$(function () {
// If "Send" button is pressed
$("#submit").click(function () {
// Get value
var name = $("#").val();
// Get email
var email = $("#email").val();
// Get comment text
var comment = $("#comment").val();
$("#answer").remove();
$("#error").remove();
if (name == "" || email == "" || comment == "") {
$("#contactform").append("<strong>Error:</strong> Fill all values.");
$("#error").hide().fadeIn();
return false;
}
$("#loading").show();
$.ajax({
type: "POST",
url: "index.php",
data: "name=" + name + "&l=" + email + "&comment=" + comment,
error: function () {
$("#contactform").append(
"<strong>Error</strong> Correct This."
);
$("#error").hide().fadeIn();
$("#loading").fadeOut();
},
success: function (rezultat) {
$("#contactform").append(rezultat);
$("#answer").hide().fadeIn();
$("#loading").fadeOut();
},
});
return false;
});
});
Finally our CSS styles (#loading and #error classes are very important)
* {
margin: 0;
padding: 0
}
body {
font: 100% Verdana, Arial, Helvetica, sans-serif;
background: #EEE
}
#contactform {
background: #FFF;
margin: 50px auto;
width: 300px;
border: 1px solid #DDD;
padding: 30px
}
input {
margin-bottom: 10px
}
label, textarea {
display: block
}
label, input, textarea, p {
font: 0.75em Verdana, Arial, Helvetica, sans-serif;
color: #333
}
input, textarea {
padding: 5px 2px;
border: 1px solid #CCC;
width: 294px
}
textarea {
height: 100px;
line-height: 1.6em
}
input:focus, textarea:focus {
border: 1px solid #AAA
}
label {
margin-bottom: 2px;
color: #069;
font-size: 0.875em
}
#submit {
width: 100px;
margin-top: 10px
}
#submit:hover {
border: 1px solid #AAA
}
#loading {
display: none
}
#error {
font-size: 0.6875em;
background: #FFEBE8;
border: 1px solid #C33;
color: #333;
display: block;
padding: 5px
}
#answer {
font-size: 0.6875em;
background: #FFFFE0;
border: 1px solid #E6DB55;
color: #333;
display: block;
padding: 5px
}