This article demonstrates how to create fun JavaScript graphs with the chart.js library. We include examples and code to implement a graph. We also explain what data visualization is. For this example, we will use the Chart.js library, which you can find in many dashboards and graphs.
What is data visualization?
Data visualization is expressed as the application converts data into a visual context, such as a graph. A graph makes it easier for the human brain to understand and gain insight into the data. It refers to techniques to make data understandable by encoding them as visual objects (dots, lines, or bars). With those techniques, the interpretation of data can be beneficial in many ways.
High-quality rendered graphics are 30 times more likely to be read than average. In addition, the human brain processes visual data approximately 50,000 times faster. Likewise, data presented to the users with a graph is 97% more convincing.
With the short explanation of data visualization, we can now start learning how to create a graph with the chart.js library.
What is Chart.js?
There are many data visualization libraries for web developers to use. However, in this article, we will prefer Chart.js. We chose Chart.js because it’s highly customizable with many options, and is used frequently on the web.
In short, the Chart.js library can be thought of as a web tool that offers interactive charts while having advanced features yet being easy to use. You can create many graphs such as bar, line, area, and scales from this open-source data visualization library.
How to create a chart with Chart.js?
- How to install Chart.js
- Creating a canvas
- Preparing the data
- Creating the canvas and chart variables
- Customizing the chart options
- Using HTML to deploy the graph
- Rendering the graph
How to install Chart.js
To install Chart.js, it requires simply placing a script tag between the <head>
element in the HTML. We will be using version 3.7.0, as seen in the script source below.
<script src="https://cdnjs.cloudflare.com/ajax/libs/Chart.js/3.7.0/chart.min.js" ></script>
Once you include the script, we can create a simple pie chart with the steps below.
Creating a canvas
The <canvas>
element allows graphics drawn by JavaScript to display on HTML. It creates a drawing board for items that need to be visually present on the web page.
<canvas id="chartName" width="500" height="500"></canvas>
For example, we created a canvas (drawing area) with a height and width of 500px and an id of id="chartName"
in the above structure.
Preparing the data
We’ve turned a small data set into the table below to illustrate a data set example. The table includes cryptocurrencies and the market sizes of these coins.
Coin Name | Market Cap |
Bitcoin | $957 B |
Ethereum | $480 B |
Cardano | $51 B |
Ripple | $43 B |
To visualize a Chart.js graph with the data set we created, we need to prepare it with JavaScript. For this, let’s create two arrays named coins and market sizes:
// Data sizes are provided by creating an array with let
let coins = ['Bitcoin', 'Ethereum', 'Cardano', 'Ripple'];
let marketCap = [957, 480, 51, 43];
Creating the canvas and chart variables
To bring all the components together, we first need to define a variable called canvas with the value being the canvas element. We use let again for this (the getElementById
must be the same as the canvas id in the HTML element above).
let canvas = document.getElementById(‘chartName’);
Now it’s time to combine the pieces by creating a new chart that uses the canvas variable. We will also define the chart type.
// In the type section, options such as line, bar, radar,
// donut and pie, polar area, bubble and scatter can be used.
let firstChart = new Chart(canvas, {
type: 'pie',
data: {}
});
At this point, you should choose a chart type that you think will be appropriate for the data used. For example, we used a pie chart to see the distribution of coins among themselves in the data set we created. We set type to pie like below:
type: 'pie',
There are additional chart types such as:
- line
- bar
- radar
- doughnut and pie
- polar area
- bubble
- scatter
Customizing the chart options
After the chart structure is created, the Chart.js library allows many customizations. For example, you can change the colors of the graph, titles, legends, and more. This is shown in the code snippets below:
Data color:
//you can choose colors as hex code or rgb
backgroundColor: [
"#26b99a",
"#9b59b6",
"#8abb6f",
"#bfd3b7"
],
Legend options:
options: {
plugins: {
legend: {
title: {
display: true,
text: "Percentage distributions of the most known coins"
}
}
}
}
Border options:
borderColor: [
"#26b99a",
"#9b59b6",
"#8abb6f",
"#bfd3b7"
]
With so many changes you can make, each chart can be unique.
Using HTML to deploy the graph
When deploying the chart we created to the web environment, it should be noted that the <canvas>
element is placed inside the <body>
element. In addition, after the Chart.js library script is placed in the head, the following structure is formed.
<!DOCTYPE html>
<html>
<head>
<title>My First Chart</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/Chart.js/3.7.0/chart.min.js" > </script>
</head>
<body>
<div style="width:400px; height:400px;">
<canvas id="chartName"></canvas>
</div>
</body>
</html>
Similarly, the following code structure is formed when you bring together the components of the chart structure created with JavaScript.
let coins = ["Bitcoin", "Ethereum", "Cardano", "Ripple"];
let marketCap = [957, 480, 51, 43];
let canvas = document.getElementById("chartName");
let firstChart = new Chart(canvas, {
type: "pie",
data: {
labels: coins,
datasets: [
{
label: "Coin",
data: marketCap,
backgroundColor: ["#26b99a", "#9b59b6", "#8abb6f", "#bfd3b7"],
borderColor: ["#26b99a", "#9b59b6", "#8abb6f", "#bfd3b7"]
}
]
},
options: {
plugins: {
legend: {
title: {
display: true,
text: "Percentage distributions of the most known coins"
}
}
}
}
});
Rendering the graph
To render the graph now that the HTML and JavaScript are finished, combine the two. As you see below, the JavaScript is placed into a new script element in the body below the canvas. This code is now ready to be used on a webpage. Check below for a rendered image of the pie chart.
<!DOCTYPE html>
<html>
<head>
<title>My First Chart</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/Chart.js/3.7.0/chart.min.js"> </script>
</head>
<body>
<div style="width:400px; height:400px;">
<canvas id="chartName"></canvas>
</div>
<script>
let coins = ["Bitcoin", "Ethereum", "Cardano", "Ripple"];
let marketCap = [957, 480, 51, 43];
let canvas = document.getElementById("chartName");
let firstChart = new Chart(canvas, {
type: "pie",
data: {
labels: coins,
datasets: [{
label: "Coin",
data: marketCap,
backgroundColor: ["#26b99a", "#9b59b6", "#8abb6f", "#bfd3b7"],
borderColor: ["#26b99a", "#9b59b6", "#8abb6f", "#bfd3b7"]
}]
},
options: {
plugins: {
legend: {
title: {
display: true,
text: "Percentage distributions of the most known coins"
}
}
}
}
});
</script>
</body>
</html>
The code renders the following graph:
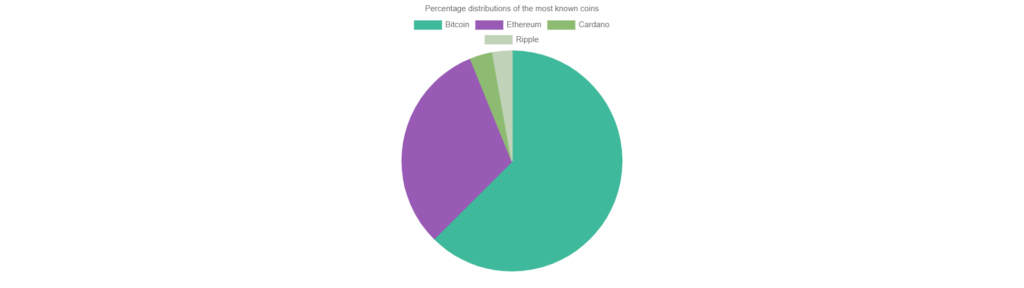
You can edit this graph example on codepen.io
Final Words
There are also libraries such as Chartist.js and Apache Echart developed for data visualization. These libraries are also JavaScript, just like Chart.js, and they are created with similar structures. But if you find other graphs for your web page that you think will increase user interaction more, the different libraries may be suitable for you.