This article demonstrates how to change the text color during hover with jQuery. Included are usable example code and a CDN link for jQuery.
This article was first seen on and republished from csstutorial.io
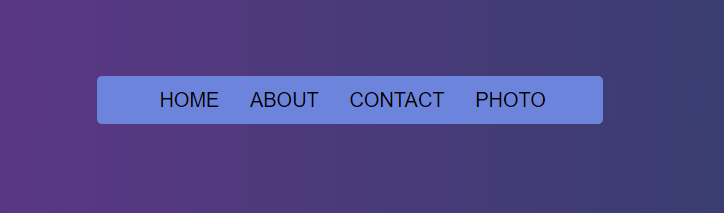
HTML
The HTML provided below constructs a simple HTML menu with four menu items.
<ul class="menu">
<li>HOME</li>
<li>ABOUT</li>
<li>CONTACT</li>
<li>PHOTO</li>
</ul>
CSS
This CSS code gives the menu structure and visibility for this example.
body,
html {
font-family: Roboto, arial, sans-serif;
background: rgb(28, 65, 97);
background: linear-gradient(
90deg,
rgba(28, 65, 97, 1) 0%,
rgba(123, 49, 150, 1) 0%,
rgba(28, 65, 97, 1) 100%
);
}
.active {
color: white;
}
ul {
list-style: none;
background: #6b83de;
}
ul li {
cursor: pointer;
display: inline-block;
margin: 10px;
}
.menu {
width: 365px;
margin: 10% auto 0 auto;
border-radius: 4px;
}
Google CDN:
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
</head>
Including jQuery library from the google CDN provides a benefit. Many users visit sites that already include this library from the Google CDN. As a result, the library is already cached in your browser whenever you visit a new website with this script included. This ultimately provides faster downloading of the web page.
jQuery
This jQuery code adds a class to the element on hover and removes the class whenever the cursor leaves the element.
$('.menu li').hover(function() {
$(this).addClass('active');
},
function() {
$(this).removeClass('active');
});
View the code example on codepen.io